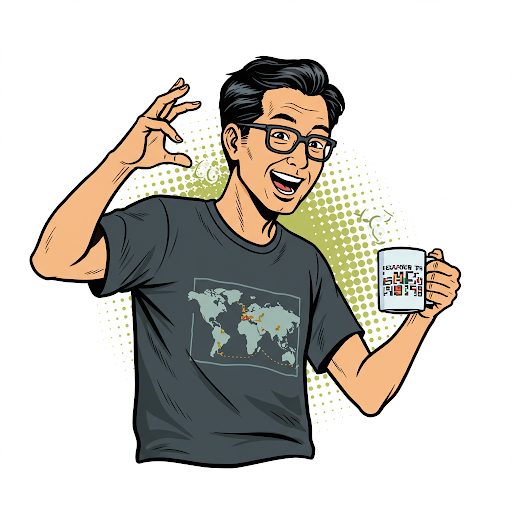
PySimpleGUIでは、タイマーストップアプリも自作できます。
公式クックブックにデモが載っています。
この記事では、公式クックブックに載っている、タイマーストップアプリを自作する方法を紹介します。
この記事に載っているサンプルコードをコピーして、Pythonで実行すれば、簡単に動きを確認できますので、是非試してみて下さい。
この記事では、初心者にもわかりやすいように、各処理の内容を、サンプルコード内にコメントとして載せています。
今回作成するアプリ
今回のアプリは、タイマーストップアプリです。
画面に、時間と、3つのボタンが表示されます。
RUN/Pause:時間計測を開始/停止します
Reset:時間をリセットします
Exit:このアプリを終わります
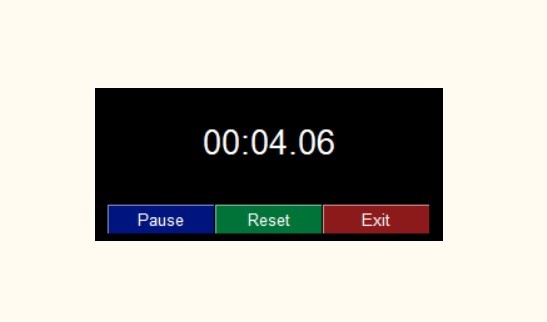
準備
PySimpleGUIのインストールの仕方は、以下の記事で紹介しています。
サンプルコード
# https://github.com/PySimpleGUI/PySimpleGUI/blob/master/DemoPrograms/Demo_Desktop_Widget_Timer.py
#!/usr/bin/env python
import PySimpleGUI as sg
import time
"""
Timer Desktop Widget Creates a floating timer that is always on top of other windows You move it by grabbing anywhere on the window Good example of how to do a non-blocking, polling program using PySimpleGUI
Something like this can be used to poll hardware when running on a Pi
While the timer ticks are being generated by PySimpleGUI's "timeout" mechanism, the actual value
of the timer that is displayed comes from the system timer, time.time(). This guarantees an
accurate time value is displayed regardless of the accuracy of the PySimpleGUI timer tick. If
this design were not used, then the time value displayed would slowly drift by the amount of time
it takes to execute the PySimpleGUI read and update calls (not good!)
Copyright 2021 PySimpleGUI
"""
# 現在時間を取得
def time_as_int():
return int(round(time.time() * 100))
# ---------------- Create Form ----------------
sg.theme('Black') # ウィンドウテーマをブラックで設定
# 画面レイアウト(1段目:空白、2段目:時間、3段目:ボタン、ボタン、ボタン)
layout = [[sg.Text('')],
[sg.Text('', size=(8, 2), font=('Helvetica', 20),justification='center', key='text')],
[sg.Button('Pause', key='-RUN-PAUSE-', button_color=('white', '#001480')),
sg.Button('Reset', button_color=('white', '#007339'), key='-RESET-'),
sg.Exit(button_color=('white', 'firebrick4'), key='Exit')]]
# ウィンドウの生成
window = sg.Window('Running Timer', layout,
no_titlebar=True, # タイトルなし
auto_size_buttons=False,
keep_on_top=True,
grab_anywhere=True,
element_padding=(0, 0),
finalize=True,
element_justification='c',
right_click_menu=sg.MENU_RIGHT_CLICK_EDITME_EXIT)
# 変数の初期化
current_time, paused_time, paused = 0, 0, False
start_time = time_as_int() # 開始時点の時間を保持
# メイン処理
while True:
# Pauseボタンが押されていないなら、時間を更新
if not paused:
event, values = window.read(timeout=10) # 入力イベントを監視
current_time = time_as_int() - start_time # 経過時間を算出(現在時間から開始時間を引いた時間)
else:
event, values = window.read() # 入力イベントを監視
# ウィンドウ上のボタン操作時の処理
if event in (sg.WIN_CLOSED, 'Exit'): # Exitボタン押下時は、処理終了
break
if event == '-RESET-': # Resetボタン押下時は、変数を全てクリアする
paused_time = start_time = time_as_int()
current_time = 0
elif event == '-RUN-PAUSE-': # RUN/Pauseボタン押下時の処理
paused = not paused # Pause状態を反転(TrueならFalseに、FalseならTrueに更新)
if paused: # pause状態なら、puase時間を更新
paused_time = time_as_int()
else: # not pause状態なら、経過時間計測を再開
start_time = start_time + time_as_int() - paused_time
# ウィンドウの表示を更新
window['-RUN-PAUSE-'].update('Run' if paused else 'Pause')
elif event == 'Edit Me':
sg.execute_editor(__file__)
# ウィンドウの表示を更新
window['text'].update('{:02d}:{:02d}.{:02d}'.format((current_time // 100) // 60,
(current_time // 100) % 60,
current_time % 100))
window.close()
動かし方
実行すると、下図のようなウィンドウが立ち上がります。時間カウントを始めています。
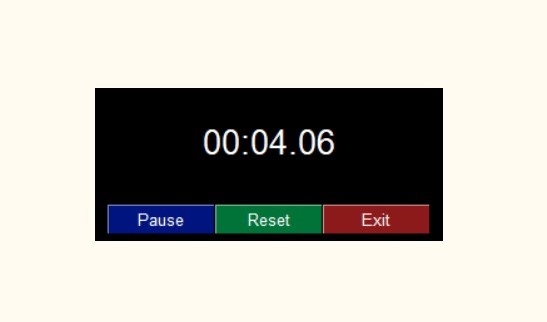
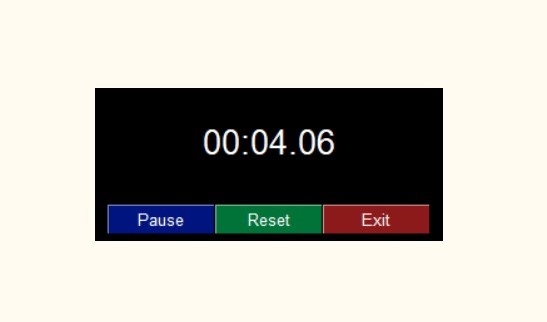
「Pause」ボタンを押すと、時間カウントが停止します。
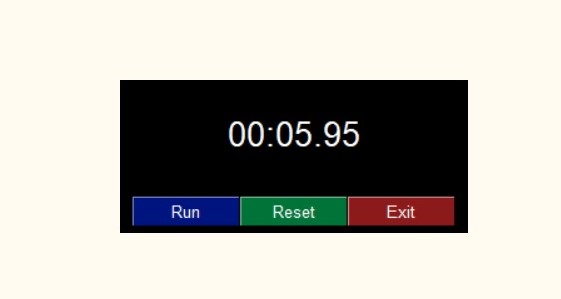
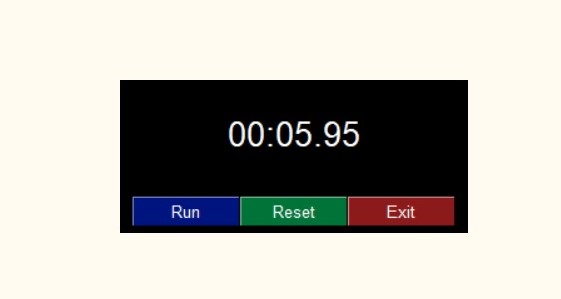
「Reset」ボタンを押すと、時間がクリアされます。
「Exit」ボタンを押すと、アプリが終了します。
まとめ
今回は、Pythonライブラリである、PySimpleGUIを使い、タイマーストップアプリの作り方を紹介しました。
M5Stack
小さな筐体の中に、ディスプレイ、ボタン、無線通信モジュールなど、必要な機能が詰め込まれたモジュールです。メーカから提供されるライブラリも豊富で、初心者の初めてのプログラミングにぴったりです。5 cm四方のベーシックなモデルの他、小型スティック型のモデル、更に小型のATOMシリーズ、M5Stampシリーズなどがあります。
AI(人工知能)特化型プログラミングスクール「Aidemy Premium」
Aidemy Premiumは、AIや機械学習などの最先端技術の修得に留まらず、それらを活用して目標達成を実現するまでを一気通貫して支援するオンラインコーチングサービスです。
こんな思いを持っている人にオススメ
・AIエンジニア/データサイエンティストにキャリアチェンジしたい
・業務課題(研究課題)をAIを使って解決したい
・教養としてAIについて知りたい
・AIに関してのスキルを身に着け就職活動に活かしたい…
オススメポイント
・3ヶ月間集中して「AIプログラミング」を修得するオンラインコーチングサービスです。
・完全なプログラミング初心者から研究者にまで幅広く優良なコンテンツを提供します。
・通常のプログラミングスクールとは異なり、受講者の進捗管理を徹底して行います。
・専属メンターがAIの学び方から徹底的にコーチングします。
・完全オンライン完結なので、東京以外に在住・在勤の方も気兼ねなくお申込み頂けます。
実績
・日本最大級のAI/人工知能プログラミングスクール
・SaaS型AI学習サービス「Aidemy」会員登録者数 55,000名突破
・法人向けAI内製化支援サービス「Aidemy Business」導入企業数 120社突破
・第16回 日本e-Learning大賞「AI・人工知能特別部門賞」受賞
・「HRアワード2019」プロフェッショナル部門 入賞
無料ビデオカウンセリング実施中です。
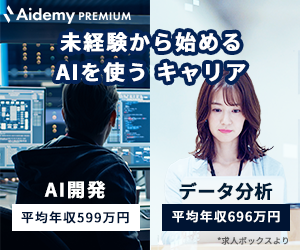
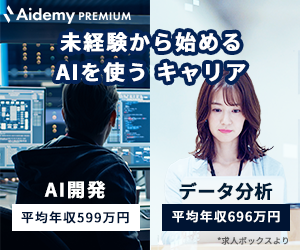

- 【PythonでGUIアプリ入門】PySimpleGUI、Pyperclip を使った クリップボードアプリ の作り方
- 【PythonでGUIアプリ入門】PySimpleGUI を使った タイマーストップアプリ の作り方
- 【Python(PySimpleGUI)でGUIアプリ入門】Pythonアプリを起動するランチャソフトの作り方
- 【PythonでGUIアプリ入門】OpenCV、PySimpleGUIによるPCカメラ映像を使った映像加工アプリの作り方
- 【PythonでGUIアプリ入門】OpenCV、PySimpleGUIで、PCカメラで撮影した映像を表示するアプリの作り方
- 【PythonでGUIアプリ入門】OpenCV、PySimpleGUIを使った、メディアプレイヤーアプリの作り方
コメント